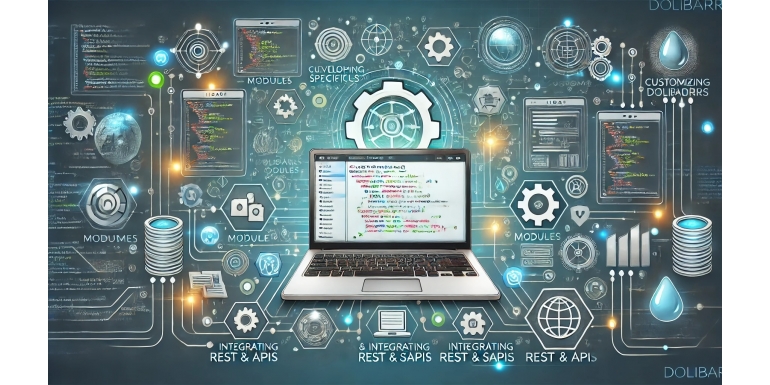
Introduction
Dolibarr is a highly popular open-source ERP/CRM solution used by SMEs and freelancers due to its flexibility and extensive functionality. However, to meet specific business needs, it is often necessary to develop custom modules and integrate Dolibarr with other systems using REST and SOAP APIs.
In this article, we will explore in detail how to customize Dolibarr by developing tailored modules and integrating third-party APIs to enhance its functionality.
1. Understanding Dolibarr’s Architecture
1.1. Modular Structure of Dolibarr
Dolibarr is built on a modular architecture, allowing users to add, activate, or deactivate features as needed. Each module is designed to be independent, which facilitates development and maintenance.
1.2. Technologies Used
Dolibarr is developed in PHP and uses a MySQL/MariaDB database. Its architecture follows an MVC (Model-View-Controller) approach, allowing a clear separation between presentation logic and business logic.
2. Developing Custom Modules
2.1. Why Develop a Custom Module?
Developing a custom module is often necessary to:
-
Add new features not included by default in Dolibarr.
-
Automate internal processes.
-
Improve the user experience with custom interfaces.
-
Integrate Dolibarr with other internal company tools.
2.2. Creating a Dolibarr Module
2.2.1. Module Structure
A Dolibarr module follows a well-defined structure:
-
mod_modulename.class.php: Defines the module's basic parameters.
-
core/modules/mod_modulename.php: Contains the module configuration.
-
class/modulename.class.php: Manages database interactions.
-
pages/modulename.php: Handles display and user interaction.
2.2.2. Example of Module Development
Creating a basic module file:
<?php
class mod_mymodule {
var $numero = 1000;
var $nom = "MyModule";
function __construct() {
global $langs;
$langs->load("mymodule@MyModule");
$this->description = $langs->trans("My custom module for Dolibarr");
}
}
?>
3. Integrating REST and SOAP APIs into Dolibarr
3.1. Why Integrate APIs into Dolibarr?
Integrating REST and SOAP APIs into Dolibarr allows users to:
-
Exchange data with third-party applications (CRM, ERP, e-commerce, etc.).
-
Automate data synchronization.
-
Develop custom connectors for specific needs.
3.2. REST API in Dolibarr
Dolibarr offers a native REST API, allowing access to data via HTTP requests.
3.2.1. Enabling the REST API
To enable the REST API in Dolibarr:
-
Go to Configuration > Modules/Applications.
-
Enable the REST API module.
-
Generate an API key for authentication.
3.2.2. Example REST API Request
Retrieve a list of customers using curl:
curl -X GET "https://example.com/api/index.php/customers" -H "DOLAPIKEY: your_api_key"
3.3. SOAP API in Dolibarr
Dolibarr also supports SOAP services for data exchange.
3.3.1. Enabling the SOAP API
-
Go to Configuration > Modules/Applications.
-
Enable the SOAP API module.
-
Configure access permissions.
3.3.2. Example of Using the SOAP API
Here is an example of a SOAP request in PHP to retrieve a customer:
$client = new SoapClient("https://example.com/api/server.php?wsdl");
$params = array('DOLAPIKEY' => 'your_api_key', 'id' => 1);
$response = $client->getCustomer($params);
print_r($response);
4. Best Practices for Development and Integration
4.1. Securing API Access
-
Use API keys and encrypted protocols (HTTPS).
-
Limit permissions for users accessing the APIs.
4.2. Documentation and Maintainability
-
Document the code and API endpoints.
-
Create logs to track interactions with APIs.
4.3. Testing and Validation
-
Test modules in a development environment before deploying to production.
-
Use tools like Postman to test API requests.
Conclusion
Customizing Dolibarr through the development of tailored modules and integrating REST and SOAP APIs allows the ERP to be adapted to the specific needs of your business. By following best practices for development and security, you ensure a stable, high-performance system that seamlessly integrates with your other IT tools. With this knowledge, you can transform Dolibarr into a fully customized solution tailored to your business activities.